As I mentioned in my first article on setting up Python, some python modules require a C/C++ compiler to build. In many cases, the developers of such modules will provide an .exe installer for Windows users, but if you have a compiler set up, you can simply download and install the modules using pip, which is much quicker and easier. Unfortunately, Windows does not come with a built-in compiler, so you have to install one.
Visual Studio Express
Visual Studio Express is the free version of Visual Studio, Microsoft's IDE and compiler suite. It's easy to setup. Just go to http://www.visualstudio.com/en-us/downloads/download-visual-studio-vs, and install the latest version of Visual Studio Express for Windows Desktop. At the time of writing, Visual Studio Express 2013 is available on the downloads page. I currently use the 2012 edition, which doesn't seem to be available any more.
After 30 days, you will be required to register the program, but don't worry - it will remain free.
When you install Visual Studio Express 2013, an environment variable called VS120COMNTOOLS will be added to your system. You can check the environment variable has been added by opening the Command Prompt, typing "set", and hitting enter.

Unfortunately, the Python package distribution module, distutils, only recognizes the version of Visual Studio used to build your Python interpreter. Currently, the official Python interpreters on python.org were made with Visual Studio 2008 (version 9.0), so when you try to build a module, distutils looks for an environment variable called VS90COMNTOOLS. Luckily, there is a very simple work-around. I can't guarantee it will work all of the time, but it has worked for me.
Simply create a VS90COMNTOOLS environment variable, and point it to your VS120COMNTOOLS. There is a good explanation of how to add an environment variable at https://kb.wisc.edu/cae/page.php?id=24500. In our case, the Variable Name should be VS90COMNTOOLS, and the Variable Value should be %VS120COMNTOOLS%. (see below)
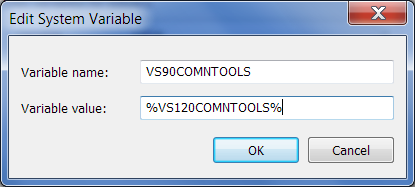
Build Your First Python Module with a C Extension
Once you've made a VS90COMNTOOLS environment variable that points to %VS120COMNTOOLS%, distutils will automatically use your compiler when you build modules using setup.py or pip install. To see if everything is working, let's try to install pyCrypto, a popular cryptography module
First open a Visual Studio command prompt. The Visual Studio command prompts are command prompts with compilers added to the path. You can find them in the the start menu under All Programs > Microsoft Visual Studio 2013. Open the VS command prompt for your system architecture (x64 Cross Tools or x86 Native Tools for 64-bit or 32-bit, respectively). Then simply type "pip install pyCrytpo". If everything is set up properly, the package will download, compile, and install automatically. You'll see a bunch of compiler options whizzing up your screen, like in the image below.

To quickly test the module, start python in interactive mode (type "python" on the command line) and enter "import Crypto". If nothing happens, the module has been installed successfully. Congratulations, you compiled a program!
Wheels and eggs
Unfortunately, there are some modules that are just too much trouble to compile on Windows, usually because they require 3rd party libraries. Fortunately, many developers provide pre-compiled binaries in the form of "wheels" or "eggs". Eggs can be installed with easy_install (which is part of the setuptools module). To download and install an egg, simply type "easy_install" followed by the name of the module. For example, "easy_install zope.interface" will download and install the egg for the zope.interface module.
However, a supposedly better (though I don't know why - it's beyond my technical understanding) method is to install a wheel. To install wheels, you first need the wheel module (of course, another module...). On your command line enter "pip install wheel", and the module will be downloaded and installed. Now you can install wheels with pip by adding the --use-wheel switch. PyZMQ is a popular module that is distributed as a wheel. To try install it, enter on your command line "pip install --use-wheel pyzmq". (see below)
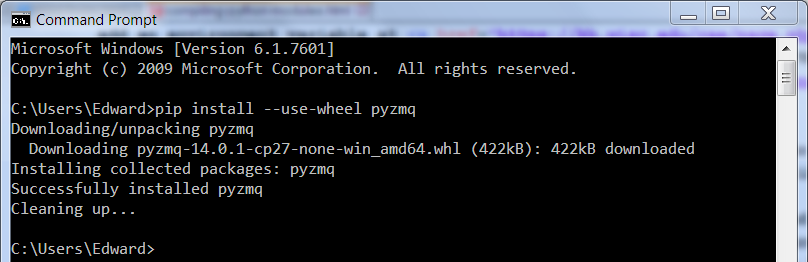
Standalone EXEs
There are a few modules that can't easily be compiled on Windows, and are not distributed as wheels or eggs. In these cases, you will have to download an exe or msi and manually go through the setup wizard. You can usually find an exe by going to the official Python package index(a.k.a. pypi), and searching for the module you need. You will be able to either download the executable directly from the module's page on pypi, or there will be a link to a download page. Try searching pypi for lxml and installing the exe (at time of writing, version 3.2.5 and 3.3.0 beta are available on pypi). It's a great module that drastically simplifies working with xml and html.
Whenever you go to download a module, I recommend using your Visual Studio command promp, so the compiler is ready. I've developed a sort of order of operations when trying to install modules. First I try pip (e.g. pip install module). That usually works, but if it doesn't, I try "pip install --use-wheel module". If that fails, open a non-visual studio command prompt and try easy_install (e.g. easy_install module). Finally, if all else fails, go to pypi, search for the module, and download and run its setup.exe installer.
In my next article, I'll explain how to setup NumPy, SciPy, matplotlib, and IPython, but I think you should now know everything you need to know to begin a module-downloading binge. Here's a list of some very useful modules:
- requests
- xlrd
- xlwt
- xlutils
- virtualenv
- nose
- lxml
- Cython
- paramiko
- pytz
Happy hacking.