About a year ago I decided to teach myself some programming. Having heard of Python from my boss, who is a programmer, I decided it was the language I'd learn. I managed to find most of the information I needed on Google, but the tutorials and how-to guides I found always seemed to miss something. An article might recommend installing a certain module, but not explain how to install modules - or fail to mention that most Python modules are distributed as .tar.gz archives, which Windows users can't open.
None of the problems were very difficult to solve, but I had to spend an unfortunate amount of time digging through forums and tutorials to find the answers. In this article, I'll try to explain everything a non-programmer needs to know to start programming with Python: installing the interpreter, adding it to your path, installing a package, and more.
The Interpreter
When someone - say, a well-meaning colleague with 20 years of programming experience - says, "just install Python, it's easy," what he really means is: "Download and install the Python interpreter and standard library." The interpreter is the program that converts the Python code you write into a kind of binary code your computer understands. The standard library is a collection of tools that let you easily do things that the interpreter can't do on its own. The individual tools are known as "modules" or "libraries". I'll explain a bit more about modules later.
So, to install Python, go to the official Python release page and choose the most recent stable version. Python 2 and Python 3 are not compatible. I currently use Python 2, because some of the modules I use require it. You might want to try the most recent release of version 2, as there are more resources on the web dedicated to it. Once you're comfortable with the basics, you can upgrade to 3. At the time of writing, the latest stable version of Python 2 is 2.7.5.
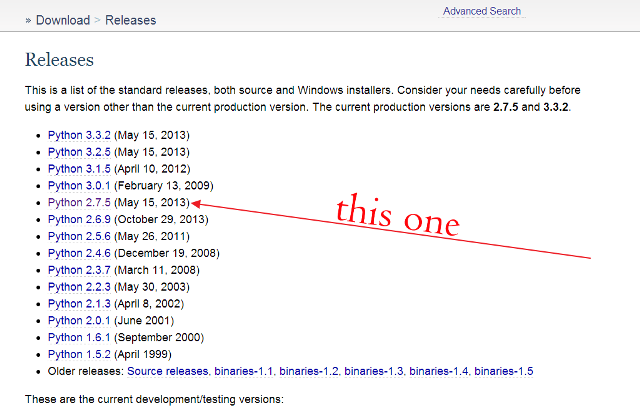
Once you select the version you want (i.e. 2.7.5), scroll down to the list of downloads and choose one of the Windows MSI Installers. If you have a 32-bit version of Windows, choose the one called "Windows x86 MSI Installer". If you have a 64-bit version of Windows, download "Windows X86-64 MSI Installer". Confusingly, the 32-bit processor architecture is known as x86, while 64-bit architecure is known as either amd64, x64, or x86_64. An entire article could be written explaining why, but the important thing to know at this point is that if you have a 32-bit computer, the programs you need as you get set up will probably mention "x86".
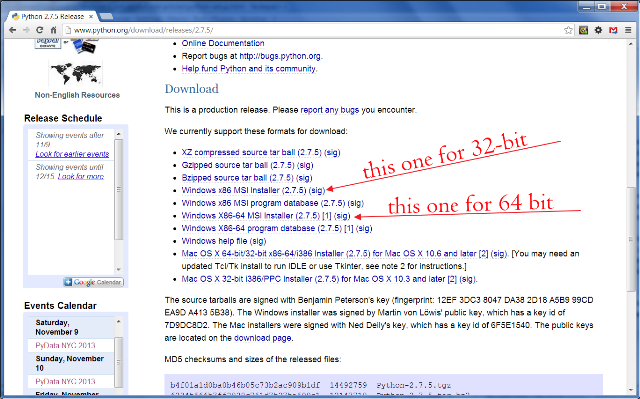
If you don't know whether your computer is 32- or 64-bit, open the Start menu and type "system". Select "System" under Control Panel. Your system type will be listed here.
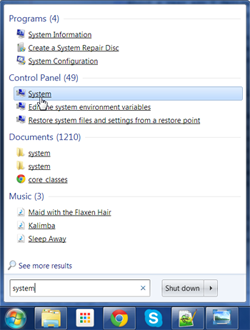
Now for the easy part. Download the installer, run it, click next a few times, and you've got Python. By default, it will be installed in the root directory of your C drive, C:\Python27 (in other words, if you open "your computer" and click on your hard drive, you should see a folder called Python27.)
Adding Python to your Path
If want to learn to program, you'll have to get used to using a command line interpreter (CLI), one of those things that look like MS-DOS. On Windows, the command line interpreter is called the Command Prompt, or cmd.exe. Open the start menu and type "command prompt" and it should be one of the top results.
To run a Python program, you would usually type "python" followed by the name of the program file. Python program files end with the extension ".py", so the program name might be something like "myprogram.py," and the full command would be "python myprogram.py". To run Python in interactive mode, you would just type "Python" and hit enter. However, if you try those commands now, you'll get an error message.
First, you need to add Python to the path. The path is simply a list of folders (or "directories", as real programmers say). Whenever you enter a command, the operating system searches through the directories in the path to find any ".exe" files that match the command you entered. So when you type "python" on the command line and hit enter, the system checks each directory in the path for a file called "python.exe."
Let's add Python to your path. Open the start menu and type "environment variables" in the search box. Choose "Edit environment variables for your account."
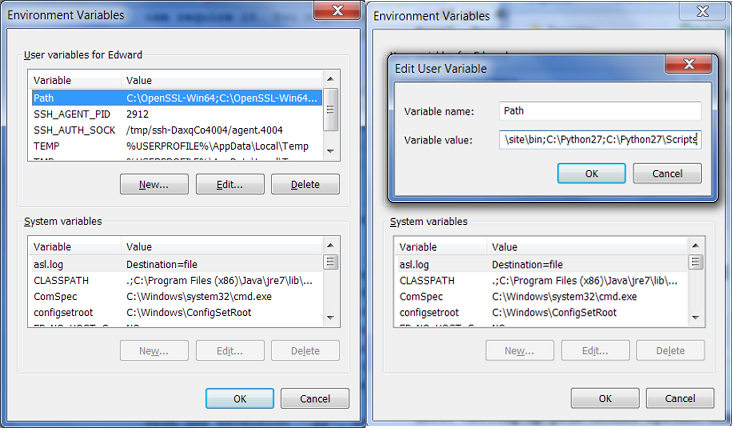
You should see the above window. This will allow you to edit the path for your user account only, so you don't have to worry about messing up your entire system. Select the item in the list called "Path" and click the Edit button below. In the "Variable value" box, add ";C:\Python27;C:\Python27\Scripts" to the end of the text.
If you don't have a Path variable, click the new button. Type "Path" for the name and "C:\Python27;C:\Python27\Scripts" for the value.
Click okay a few times. Open a new command prompt, and type "python". If everything went according to plan, you should see the interactive interpreter. Congratulations. You're a hacker.
Packages
I really wish someone had explained packages to me earlier. As soon as you start searching the web for advice on Python, you'll find people recommending all kinds of libraries and modules, but as you go to download them, you'll be caught in an endless quest to find all of the dependencies you need. Each package will seem to require some other package. And what do you do with a package you download? Where do you start?
Most Python packages can be found in the official Python Package Index, pypi.python.org. When you go to download a package, you'll probably notice that it's in .tar.gz format. This is not actually a type of file, but a type of compression, like zip. Unix-based PCs (Linux and Mac OS X) have built-in tools for decompressing .tar.gz archives, but if you have Windows, you need some new software. I recommend PeaZip, which is free.
Once you have PeaZip, go to the Python package index and search for "setuptools". Setuptools is a module that includes a package downloading program called easy_install. It is also a requirement for the popular package management program called pip. Scroll down to the installation instructions, right click on the link to ez_setup.py, and click "save link as"
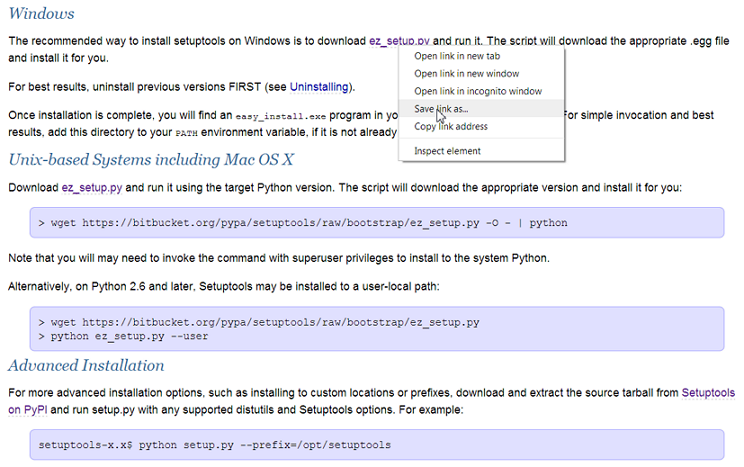
Then go to the folder where you downloaded ez_setup.py, and double-click the file. This will download and install setuptools.
Now search for "pip" in the package index. Click the large green download button on the pip page. After it downloads, open the folder where it was saved.
This is where you'll use PeaZip to extract the contents of the tar.gz archive. A tar.gz archive, sometimes called a tarball, is actually a compressed file within a compressed file - a .tar within a .gz - so you must first extract the .tar from the .gz before extracting the content of the .tar.
To extract the .tar from the .gz, right click the archive, select PeaZip, and then select "Extract here". It will create a folder called "dist". Open the folder. It should contain the .tar archive. Right click on it and select "Extract here" again.

Now, that the pip source folder has been extracted, you can install the package. To install the package, first open your Command Prompt. Type "cd" followed by the directory where pip was extracted. Cd stands for "change directory". In my case, I have to type "cd downloads\dist\pip-1.4.1".
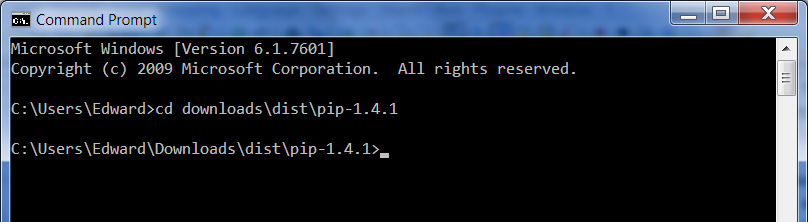
To see a list of files and subdirectories, type "dir". You should see a file called "setup.py". When a python developer decides to distribute a program or module, he will generally include a setup.py installation script. Setup.py is the standard name given to the script that invokes a module called "distutils", which is part of the standard library.
All you have to do is is type "python setup.py install" and hit enter. There are several other words that can be used after setup.py, but I won't go into that now. However, now that you have pip, installing packages is even easier.
Pip is known as a "package management" program. It takes all the stress out of installing packages, because it downloads, extracts and installs them in a single step. Better yet, it will automatically download and install any prerequisites.
One of my favorite Python libraries is called Requests. It's a module for making web requests more easily than you can with the standard library. You can install it with pip by simply typing (in the command line) "pip install requests" and hitting enter. Sometimes you'll still need to install packages manually with setup.py, and sometimes you'll need to find an installer to get a package to work on windows, but you can usually use pip. Some packages can be setup with pip only if you have a C/C++ compiler, which I'll write about another time.
And that's pretty much everything you need to know to start using Python on Windows. If you have a Macintosh or Linux PC, you're in luck because Python comes with the OS. Happy hacking.